Create your First Silverlight Application using LINQ and Web Services
Create your First Silver light Application using LINQ and Web Services
1. Create a New Silverlight Application project and name it “ImageViewer”
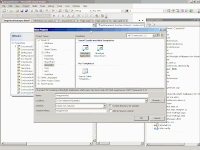
2. Select ASP .NET Web Application Project and change the name for the web project to “ImageViewer_Web”
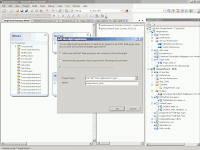
3. The solution includes two projects. One is for the Silverlight Control [i.e. ImageViewer] and Another one is for the ASP .NET web system [i.e ImageViewer_Web]
4. Now, we have to create a data context using LINQ to SQL features in ImageViewer_Web project. To do that, right click on the Web Project and Select Add->New Item and Select LINQ to SQL Classes
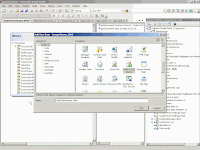
5. Then Open Server Explorer (View->Server Explorer). Create a connection to the “ImageViewer” database and drag and drop the Tables (tblIndex, DocType, tblDoc) into the left pane and Stored Procedures (getDocuments(), getDocumentTypes()) into the right pane of ImgDataManager.dbml
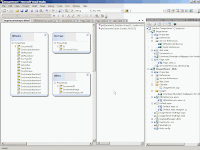
6. Name the data context as “ImgDataManagerDataContext” and set Serialization mode to Unidirectional
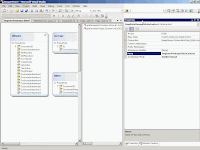
7. If you open up Data Model’s designer.cs file you will notice that it already have created the references to the tables and stored procedures that you dragged and dropped into the data model designer
8. Now Create a new Web Service DBWebService.asmx
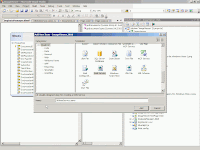
9. Then Open DBWebService.asmx.cs file and add the Web Methods to retrieve Table/Views/Stored Procedures that you added in section 5
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Xml.Linq;
namespace ImageViewer_Web
{
///
/// Summary description for DBWebService
///
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class DBWebService : System.Web.Services.WebService
{
[WebMethod]
public string myDocumentsList(string id, int docTypeId, string docHeaderNo, string sapOrderNo, string yearValue,string monthValue)
{
string subMitter = "";
ImgDataManagerDataContext db = new ImgDataManagerDataContext();
var documentList = db.getDocuments(id, docTypeId, docHeaderNo, sapOrderNo, subMitter, yearValue, monthValue);
XDocument xDoc = new XDocument(new XDeclaration("1.0", "utf-8", "yes"), new XElement("Documents", from c in documentList select new XElement("Docs", new XAttribute("CreateDate", c.CreateDate.ToString()), new XAttribute("DocumentNumber", c.DocHeaderNumber.ToString()), new XAttribute("DocumentNumber2", c.DocHeaderNumber2.ToString()), new XAttribute("DocumentNumber3", c.DocHeaderNumber3.ToString()), new XAttribute("DocumentNumber4", c.DocHeaderNumber4.ToString()), new XAttribute("DocumentNumber5", c.DocHeaderNumber5.ToString()), new XAttribute("DocumentNumber6", c.DocHeaderNumber6.ToString()), new XAttribute("DocumentNumber7", c.DocHeaderNumber7.ToString()), new XAttribute("DocumentNumber8", c.DocHeaderNumber8.ToString()), new XAttribute("DocTypeID", c.DocTypeID.ToString()), new XAttribute("DocumentID", c.DocumentID.ToString()), new XAttribute("YearValue", c.YearValue.ToString()), new XAttribute("MonthValue", c.MonthValue.ToString()), new XAttribute("SAPNumber", c.SAPNumber.ToString()), new XAttribute("SAPNumber2", c.SAPNumber2.ToString()), new XAttribute("Submitter", c.Submitter.ToString()), new XAttribute("DocumentImage", c.DocumentImage.ToString()))));
//return
return xDoc.ToString();
}
[WebMethod]
public string myDocumentTypesList()
{
ImgDataManagerDataContext db = new ImgDataManagerDataContext();
var documentList = db.getDocumentTypes(null);
XDocument xDocType = new XDocument(new XDeclaration("1.0", "utf-8", "yes"), new XElement("DocumentTypes", from c in documentList select new XElement("Types", new XAttribute("DocTypeID", c.ID), new XAttribute("DocumentType", c.DocumentType.ToString()))));
//return
return xDocType.ToString();
}
}
}
10. Now create a reference to this web service from the Silverlight Project. Right Click on the Project [i.e. ImageViewer] and Add Service Reference.
Then, click on discover and select DBWebService and name it as DBWebSrvReference
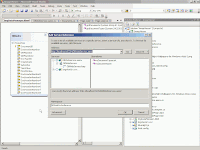
It will add the service reference to your ServiceReferences.ClientConfig file
11. Now, we will create a Data layer class to access the data through web services
using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace ImageViewer
{
public class Documents
{
public string CreateDate { get; set; }
public string DocumentNumber { get; set; }
public string DocumentNumber2 { get; set; }
public string DocumentNumber3 { get; set; }
public string DocumentNumber4 { get; set; }
public string DocumentNumber5 { get; set; }
public string DocumentNumber6 { get; set; }
public string DocumentNumber7 { get; set; }
public string DocumentNumber8 { get; set; }
public string DocumentImage { get; set; }
public string DocTypeID { get; set; }
public string DocumentID { get; set; }
public string MonthValue { get; set; }
public string YearValue { get; set; }
public string SAPNumber { get; set; }
public string SAPNumber2 { get; set; }
public string Submitter { get; set; }
}
public class DocumentTypes
{
public string DocTypeID { get; set; }
public string DocumentType { get; set; }
}
public class DropDownBox
{
private int _value;
private string _name;
public int Value
{
get { return _value; }
set { _value = value; }
}
public string Name
{
get { return _name; }
set { _name = value; }
}
public DropDownBox(int value, string name)
{
_name = name;
_value = value;
}
public override string ToString()
{
return _name;
}
}
}
12. Add a reference to System.Windows.Controls.Data to ImageViewer project.
13. Open up the Page.xaml file from Microsoft Expression Blend and add necessary controls [Right Click on the File and Choose Open in Expression Blend]
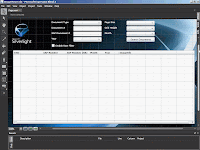
14. Page.xaml file should look like this:
15. You will notice that we have Loaded Event Handler for each Data Bind Objects [ComboBox, Grid]. Then we have to create those Loaded event handlers into the Page.xaml.cs file
16. Now add necessary functions into the Page.xaml.cs file
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using ImageViewer.ImgSrvReference;
using ImageViewer.DBWebSrvReference;
using System.Xml.Linq;
namespace ImageViewer
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
}
private void DataGrid_Loaded(object sender, RoutedEventArgs e)
{
}
void myDBSvc_GetDocumentsCompleted(object sender, ImageViewer.DBWebSrvReference.myDocumentsListCompletedEventArgs e)
{
if (e.Error == null)
{
//show data
ShowData(e.Result);
}
}
void myDBSvc_GetDocumentTypesCompleted(object sender, ImageViewer.DBWebSrvReference.myDocumentTypesListCompletedEventArgs e)
{
if (e.Error == null)
{
//show data
loadDocumentTypes(e.Result);
}
}
void ShowData(string xmlData)
{
XDocument xmlDocuments = XDocument.Parse(xmlData);
//LINQ to XML query, to extract response from Web Service
var myDocuments = from doc in xmlDocuments.Descendants("Docs")
where doc.Attribute("DocumentNumber") != null
select new Documents
{
CreateDate = (string)doc.Attribute("CreateDate"),
DocumentNumber = (string)doc.Attribute("DocumentNumber"),
DocumentNumber2 = (string)doc.Attribute("DocumentNumber2"),
DocumentNumber3 = (string)doc.Attribute("DocumentNumber3"),
DocumentNumber4 = (string)doc.Attribute("DocumentNumber4"),
DocumentNumber5 = (string)doc.Attribute("DocumentNumber5"),
DocumentNumber6 = (string)doc.Attribute("DocumentNumber6"),
DocumentNumber7 = (string)doc.Attribute("DocumentNumber7"),
DocumentNumber8 = (string)doc.Attribute("DocumentNumber8"),
DocumentImage = (string)doc.Attribute("DocumentImage"),
DocTypeID = (string)doc.Attribute("DocTypeID"),
DocumentID = (string)doc.Attribute("DocumentID"),
MonthValue = (string)doc.Attribute("MonthValue"),
YearValue = (string)doc.Attribute("YearValue"),
SAPNumber = (string)doc.Attribute("SAPNumber"),
SAPNumber2 = (string)doc.Attribute("SAPNumber2"),
Submitter = (string)doc.Attribute("Submitter")
};
//bind to DataGrid
dgIndexes.Visibility = Visibility.Visible;
//attach to DataGrid, ToList() is used to enable DataGrid sorting which needs ILIST interface
dgIndexes.ItemsSource = myDocuments.ToList();
}
void loadDocumentTypes(string xmlData)
{
XDocument xmlDocumentTypes = XDocument.Parse(xmlData);
//LINQ to XML query, to extract response from Web Service
var myDocumentTypes = from doc in xmlDocumentTypes.Descendants("Types")
where doc.Attribute("DocTypeID") != null
select new DocumentTypes
{
DocTypeID = (string)doc.Attribute("DocTypeID"),
DocumentType = (string)doc.Attribute("DocumentType"),
};
//bind to Combo
ddDocumentType.Visibility = Visibility.Visible;
//attach to DataGrid, ToList() is used to enable DataGrid sorting which needs ILIST interface
ddDocumentType.ItemsSource = myDocumentTypes.ToList();
ddDocumentType.DisplayMemberPath = "DocumentType";
ddDocumentType.SelectedIndex = 0;
}
void loadYear()
{
ddYear.Items.Clear();
ddYear.Items.Add("Show All");
for (int i = 2000; i <= 2015; i++)
{
ddYear.Items.Add(new DropDownBox(i, i.ToString()));
}
ddYear.SelectedIndex = 0;
}
void loadMonth()
{
ddMonth.Items.Add(new DropDownBox(0, "Show All"));
ddMonth.Items.Add(new DropDownBox(1,"January"));
ddMonth.Items.Add(new DropDownBox(2, "February"));
ddMonth.Items.Add(new DropDownBox(3, "March"));
ddMonth.Items.Add(new DropDownBox(4, "April"));
ddMonth.Items.Add(new DropDownBox(5, "May"));
ddMonth.Items.Add(new DropDownBox(6, "June"));
ddMonth.Items.Add(new DropDownBox(7, "July"));
ddMonth.Items.Add(new DropDownBox(8, "August"));
ddMonth.Items.Add(new DropDownBox(9, "September"));
ddMonth.Items.Add(new DropDownBox(10, "October"));
ddMonth.Items.Add(new DropDownBox(11, "November"));
ddMonth.Items.Add(new DropDownBox(12, "December"));
ddMonth.SelectedIndex = 0;
}
void loadPageSize()
{
ddPageSize.Items.Add(new DropDownBox(0, "Show All"));
ddPageSize.Items.Add(new DropDownBox(10, "10"));
ddPageSize.Items.Add(new DropDownBox(20, "20"));
ddPageSize.Items.Add(new DropDownBox(50, "50"));
ddPageSize.Items.Add(new DropDownBox(100, "100"));
ddPageSize.Items.Add(new DropDownBox(500, "500"));
ddPageSize.SelectedIndex = 0;
}
void loadGridHeight()
{
ddGridHeight.Items.Add(new DropDownBox(0, "Full Page"));
ddGridHeight.Items.Add(new DropDownBox(1, "300px"));
ddGridHeight.Items.Add(new DropDownBox(2, "500px"));
ddGridHeight.Items.Add(new DropDownBox(3, "1000px"));
ddGridHeight.SelectedIndex = 0;
}
public static string GetUrlForResource(string resourcePage)
{
string webUrl = System.Windows.Browser.HtmlPage.Document.DocumentUri.ToString();
string containerPage = webUrl.Substring(webUrl.LastIndexOf("/") + 1);
webUrl = webUrl.Replace(containerPage, resourcePage);
return webUrl;
}
private void btnSubmitSearch_Click(object sender, RoutedEventArgs e)
{
try
{
string webServiceUrl = GetUrlForResource("DBWebService.asmx");
System.ServiceModel.BasicHttpBinding binding = new System.ServiceModel.BasicHttpBinding();
binding.MaxReceivedMessageSize = int.MaxValue;
ImageViewer.DBWebSrvReference.DBWebServiceSoapClient myDBSvc = new ImageViewer.DBWebSrvReference.DBWebServiceSoapClient(binding, new System.ServiceModel.EndpointAddress(webServiceUrl));
string _Id = "";
int docTypeId = 0;
string docHeaderNo = "";
string sapOrderNo = "";
string yearValue = "";
string monthValue = "";
if (ddDocumentType.SelectedItem.ToString() != "0")
{
DocumentTypes myDocType = (DocumentTypes)ddDocumentType.SelectedItem;
docTypeId = Convert.ToInt32(myDocType.DocTypeID.ToString());
}
if (this.txtDocumentNo.Text != "")
{
docHeaderNo = this.txtDocumentNo.Text;
}
if (txtSAPNo.Text != "")
{
sapOrderNo = txtSAPNo.Text;
}
if (ddYear.SelectedItem.ToString() != "Show All")
{
DropDownBox myObject = (DropDownBox)ddYear.SelectedItem;
yearValue = myObject.Value.ToString();
}
if (ddMonth.SelectedItem.ToString() != "Show All")
{
DropDownBox myObject = (DropDownBox)ddMonth.SelectedItem;
monthValue = myObject.Value.ToString();
}
myDBSvc.myDocumentsListCompleted += new EventHandler(myDBSvc_GetDocumentsCompleted);
myDBSvc.myDocumentsListAsync(_Id, docTypeId, docHeaderNo, sapOrderNo, yearValue, monthValue);
}
catch (Exception ex)
{
lblMessage.Text = "**" + ex.Message.ToString();
}
}
private void ComboBox_Loaded(object sender, RoutedEventArgs e)
{
}
private void ddDocumentType_Loaded(object sender, RoutedEventArgs e)
{
string webServiceUrl = GetUrlForResource("DBWebService.asmx");
System.ServiceModel.BasicHttpBinding binding = new System.ServiceModel.BasicHttpBinding();
binding.MaxReceivedMessageSize = int.MaxValue;
ImageViewer.DBWebSrvReference.DBWebServiceSoapClient myDBSvc = new ImageViewer.DBWebSrvReference.DBWebServiceSoapClient(binding, new System.ServiceModel.EndpointAddress(webServiceUrl));
myDBSvc.myDocumentTypesListCompleted += new EventHandler(myDBSvc_GetDocumentTypesCompleted);
myDBSvc.myDocumentTypesListAsync();
}
private void ddYear_Loaded(object sender, RoutedEventArgs e)
{
loadYear();
}
private void ddMonth_Loaded(object sender, RoutedEventArgs e)
{
loadMonth();
}
private void ddGridHeight_Loaded(object sender, RoutedEventArgs e)
{
loadGridHeight();
}
private void ddPageSize_Loaded(object sender, RoutedEventArgs e)
{
loadPageSize();
}
void showError(string localError)
{
}
}
}
17. Then compile and run the project
18. Be sure to add .xap and .xaml MIME types after the deployment into IIR
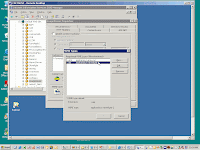
19. Now, if we run ImageViewerTestPage.aspx from the browser, we will see the Silverlight Application Interface that we created
1. Create a New Silverlight Application project and name it “ImageViewer”
2. Select ASP .NET Web Application Project and change the name for the web project to “ImageViewer_Web”
3. The solution includes two projects. One is for the Silverlight Control [i.e. ImageViewer] and Another one is for the ASP .NET web system [i.e ImageViewer_Web]
4. Now, we have to create a data context using LINQ to SQL features in ImageViewer_Web project. To do that, right click on the Web Project and Select Add->New Item and Select LINQ to SQL Classes
5. Then Open Server Explorer (View->Server Explorer). Create a connection to the “ImageViewer” database and drag and drop the Tables (tblIndex, DocType, tblDoc) into the left pane and Stored Procedures (getDocuments(), getDocumentTypes()) into the right pane of ImgDataManager.dbml
6. Name the data context as “ImgDataManagerDataContext” and set Serialization mode to Unidirectional
7. If you open up Data Model’s designer.cs file you will notice that it already have created the references to the tables and stored procedures that you dragged and dropped into the data model designer
8. Now Create a new Web Service DBWebService.asmx
9. Then Open DBWebService.asmx.cs file and add the Web Methods to retrieve Table/Views/Stored Procedures that you added in section 5
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Xml.Linq;
namespace ImageViewer_Web
{
///
/// Summary description for DBWebService
///
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class DBWebService : System.Web.Services.WebService
{
[WebMethod]
public string myDocumentsList(string id, int docTypeId, string docHeaderNo, string sapOrderNo, string yearValue,string monthValue)
{
string subMitter = "";
ImgDataManagerDataContext db = new ImgDataManagerDataContext();
var documentList = db.getDocuments(id, docTypeId, docHeaderNo, sapOrderNo, subMitter, yearValue, monthValue);
XDocument xDoc = new XDocument(new XDeclaration("1.0", "utf-8", "yes"), new XElement("Documents", from c in documentList select new XElement("Docs", new XAttribute("CreateDate", c.CreateDate.ToString()), new XAttribute("DocumentNumber", c.DocHeaderNumber.ToString()), new XAttribute("DocumentNumber2", c.DocHeaderNumber2.ToString()), new XAttribute("DocumentNumber3", c.DocHeaderNumber3.ToString()), new XAttribute("DocumentNumber4", c.DocHeaderNumber4.ToString()), new XAttribute("DocumentNumber5", c.DocHeaderNumber5.ToString()), new XAttribute("DocumentNumber6", c.DocHeaderNumber6.ToString()), new XAttribute("DocumentNumber7", c.DocHeaderNumber7.ToString()), new XAttribute("DocumentNumber8", c.DocHeaderNumber8.ToString()), new XAttribute("DocTypeID", c.DocTypeID.ToString()), new XAttribute("DocumentID", c.DocumentID.ToString()), new XAttribute("YearValue", c.YearValue.ToString()), new XAttribute("MonthValue", c.MonthValue.ToString()), new XAttribute("SAPNumber", c.SAPNumber.ToString()), new XAttribute("SAPNumber2", c.SAPNumber2.ToString()), new XAttribute("Submitter", c.Submitter.ToString()), new XAttribute("DocumentImage", c.DocumentImage.ToString()))));
//return
return xDoc.ToString();
}
[WebMethod]
public string myDocumentTypesList()
{
ImgDataManagerDataContext db = new ImgDataManagerDataContext();
var documentList = db.getDocumentTypes(null);
XDocument xDocType = new XDocument(new XDeclaration("1.0", "utf-8", "yes"), new XElement("DocumentTypes", from c in documentList select new XElement("Types", new XAttribute("DocTypeID", c.ID), new XAttribute("DocumentType", c.DocumentType.ToString()))));
//return
return xDocType.ToString();
}
}
}
10. Now create a reference to this web service from the Silverlight Project. Right Click on the Project [i.e. ImageViewer] and Add Service Reference.
Then, click on discover and select DBWebService and name it as DBWebSrvReference
It will add the service reference to your ServiceReferences.ClientConfig file
11. Now, we will create a Data layer class to access the data through web services
using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace ImageViewer
{
public class Documents
{
public string CreateDate { get; set; }
public string DocumentNumber { get; set; }
public string DocumentNumber2 { get; set; }
public string DocumentNumber3 { get; set; }
public string DocumentNumber4 { get; set; }
public string DocumentNumber5 { get; set; }
public string DocumentNumber6 { get; set; }
public string DocumentNumber7 { get; set; }
public string DocumentNumber8 { get; set; }
public string DocumentImage { get; set; }
public string DocTypeID { get; set; }
public string DocumentID { get; set; }
public string MonthValue { get; set; }
public string YearValue { get; set; }
public string SAPNumber { get; set; }
public string SAPNumber2 { get; set; }
public string Submitter { get; set; }
}
public class DocumentTypes
{
public string DocTypeID { get; set; }
public string DocumentType { get; set; }
}
public class DropDownBox
{
private int _value;
private string _name;
public int Value
{
get { return _value; }
set { _value = value; }
}
public string Name
{
get { return _name; }
set { _name = value; }
}
public DropDownBox(int value, string name)
{
_name = name;
_value = value;
}
public override string ToString()
{
return _name;
}
}
}
12. Add a reference to System.Windows.Controls.Data to ImageViewer project.
13. Open up the Page.xaml file from Microsoft Expression Blend and add necessary controls [Right Click on the File and Choose Open in Expression Blend]
14. Page.xaml file should look like this:
15. You will notice that we have Loaded Event Handler for each Data Bind Objects [ComboBox, Grid]. Then we have to create those Loaded event handlers into the Page.xaml.cs file
16. Now add necessary functions into the Page.xaml.cs file
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using ImageViewer.ImgSrvReference;
using ImageViewer.DBWebSrvReference;
using System.Xml.Linq;
namespace ImageViewer
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
}
private void DataGrid_Loaded(object sender, RoutedEventArgs e)
{
}
void myDBSvc_GetDocumentsCompleted(object sender, ImageViewer.DBWebSrvReference.myDocumentsListCompletedEventArgs e)
{
if (e.Error == null)
{
//show data
ShowData(e.Result);
}
}
void myDBSvc_GetDocumentTypesCompleted(object sender, ImageViewer.DBWebSrvReference.myDocumentTypesListCompletedEventArgs e)
{
if (e.Error == null)
{
//show data
loadDocumentTypes(e.Result);
}
}
void ShowData(string xmlData)
{
XDocument xmlDocuments = XDocument.Parse(xmlData);
//LINQ to XML query, to extract response from Web Service
var myDocuments = from doc in xmlDocuments.Descendants("Docs")
where doc.Attribute("DocumentNumber") != null
select new Documents
{
CreateDate = (string)doc.Attribute("CreateDate"),
DocumentNumber = (string)doc.Attribute("DocumentNumber"),
DocumentNumber2 = (string)doc.Attribute("DocumentNumber2"),
DocumentNumber3 = (string)doc.Attribute("DocumentNumber3"),
DocumentNumber4 = (string)doc.Attribute("DocumentNumber4"),
DocumentNumber5 = (string)doc.Attribute("DocumentNumber5"),
DocumentNumber6 = (string)doc.Attribute("DocumentNumber6"),
DocumentNumber7 = (string)doc.Attribute("DocumentNumber7"),
DocumentNumber8 = (string)doc.Attribute("DocumentNumber8"),
DocumentImage = (string)doc.Attribute("DocumentImage"),
DocTypeID = (string)doc.Attribute("DocTypeID"),
DocumentID = (string)doc.Attribute("DocumentID"),
MonthValue = (string)doc.Attribute("MonthValue"),
YearValue = (string)doc.Attribute("YearValue"),
SAPNumber = (string)doc.Attribute("SAPNumber"),
SAPNumber2 = (string)doc.Attribute("SAPNumber2"),
Submitter = (string)doc.Attribute("Submitter")
};
//bind to DataGrid
dgIndexes.Visibility = Visibility.Visible;
//attach to DataGrid, ToList() is used to enable DataGrid sorting which needs ILIST interface
dgIndexes.ItemsSource = myDocuments.ToList();
}
void loadDocumentTypes(string xmlData)
{
XDocument xmlDocumentTypes = XDocument.Parse(xmlData);
//LINQ to XML query, to extract response from Web Service
var myDocumentTypes = from doc in xmlDocumentTypes.Descendants("Types")
where doc.Attribute("DocTypeID") != null
select new DocumentTypes
{
DocTypeID = (string)doc.Attribute("DocTypeID"),
DocumentType = (string)doc.Attribute("DocumentType"),
};
//bind to Combo
ddDocumentType.Visibility = Visibility.Visible;
//attach to DataGrid, ToList() is used to enable DataGrid sorting which needs ILIST interface
ddDocumentType.ItemsSource = myDocumentTypes.ToList();
ddDocumentType.DisplayMemberPath = "DocumentType";
ddDocumentType.SelectedIndex = 0;
}
void loadYear()
{
ddYear.Items.Clear();
ddYear.Items.Add("Show All");
for (int i = 2000; i <= 2015; i++)
{
ddYear.Items.Add(new DropDownBox(i, i.ToString()));
}
ddYear.SelectedIndex = 0;
}
void loadMonth()
{
ddMonth.Items.Add(new DropDownBox(0, "Show All"));
ddMonth.Items.Add(new DropDownBox(1,"January"));
ddMonth.Items.Add(new DropDownBox(2, "February"));
ddMonth.Items.Add(new DropDownBox(3, "March"));
ddMonth.Items.Add(new DropDownBox(4, "April"));
ddMonth.Items.Add(new DropDownBox(5, "May"));
ddMonth.Items.Add(new DropDownBox(6, "June"));
ddMonth.Items.Add(new DropDownBox(7, "July"));
ddMonth.Items.Add(new DropDownBox(8, "August"));
ddMonth.Items.Add(new DropDownBox(9, "September"));
ddMonth.Items.Add(new DropDownBox(10, "October"));
ddMonth.Items.Add(new DropDownBox(11, "November"));
ddMonth.Items.Add(new DropDownBox(12, "December"));
ddMonth.SelectedIndex = 0;
}
void loadPageSize()
{
ddPageSize.Items.Add(new DropDownBox(0, "Show All"));
ddPageSize.Items.Add(new DropDownBox(10, "10"));
ddPageSize.Items.Add(new DropDownBox(20, "20"));
ddPageSize.Items.Add(new DropDownBox(50, "50"));
ddPageSize.Items.Add(new DropDownBox(100, "100"));
ddPageSize.Items.Add(new DropDownBox(500, "500"));
ddPageSize.SelectedIndex = 0;
}
void loadGridHeight()
{
ddGridHeight.Items.Add(new DropDownBox(0, "Full Page"));
ddGridHeight.Items.Add(new DropDownBox(1, "300px"));
ddGridHeight.Items.Add(new DropDownBox(2, "500px"));
ddGridHeight.Items.Add(new DropDownBox(3, "1000px"));
ddGridHeight.SelectedIndex = 0;
}
public static string GetUrlForResource(string resourcePage)
{
string webUrl = System.Windows.Browser.HtmlPage.Document.DocumentUri.ToString();
string containerPage = webUrl.Substring(webUrl.LastIndexOf("/") + 1);
webUrl = webUrl.Replace(containerPage, resourcePage);
return webUrl;
}
private void btnSubmitSearch_Click(object sender, RoutedEventArgs e)
{
try
{
string webServiceUrl = GetUrlForResource("DBWebService.asmx");
System.ServiceModel.BasicHttpBinding binding = new System.ServiceModel.BasicHttpBinding();
binding.MaxReceivedMessageSize = int.MaxValue;
ImageViewer.DBWebSrvReference.DBWebServiceSoapClient myDBSvc = new ImageViewer.DBWebSrvReference.DBWebServiceSoapClient(binding, new System.ServiceModel.EndpointAddress(webServiceUrl));
string _Id = "";
int docTypeId = 0;
string docHeaderNo = "";
string sapOrderNo = "";
string yearValue = "";
string monthValue = "";
if (ddDocumentType.SelectedItem.ToString() != "0")
{
DocumentTypes myDocType = (DocumentTypes)ddDocumentType.SelectedItem;
docTypeId = Convert.ToInt32(myDocType.DocTypeID.ToString());
}
if (this.txtDocumentNo.Text != "")
{
docHeaderNo = this.txtDocumentNo.Text;
}
if (txtSAPNo.Text != "")
{
sapOrderNo = txtSAPNo.Text;
}
if (ddYear.SelectedItem.ToString() != "Show All")
{
DropDownBox myObject = (DropDownBox)ddYear.SelectedItem;
yearValue = myObject.Value.ToString();
}
if (ddMonth.SelectedItem.ToString() != "Show All")
{
DropDownBox myObject = (DropDownBox)ddMonth.SelectedItem;
monthValue = myObject.Value.ToString();
}
myDBSvc.myDocumentsListCompleted += new EventHandler
myDBSvc.myDocumentsListAsync(_Id, docTypeId, docHeaderNo, sapOrderNo, yearValue, monthValue);
}
catch (Exception ex)
{
lblMessage.Text = "**" + ex.Message.ToString();
}
}
private void ComboBox_Loaded(object sender, RoutedEventArgs e)
{
}
private void ddDocumentType_Loaded(object sender, RoutedEventArgs e)
{
string webServiceUrl = GetUrlForResource("DBWebService.asmx");
System.ServiceModel.BasicHttpBinding binding = new System.ServiceModel.BasicHttpBinding();
binding.MaxReceivedMessageSize = int.MaxValue;
ImageViewer.DBWebSrvReference.DBWebServiceSoapClient myDBSvc = new ImageViewer.DBWebSrvReference.DBWebServiceSoapClient(binding, new System.ServiceModel.EndpointAddress(webServiceUrl));
myDBSvc.myDocumentTypesListCompleted += new EventHandler
myDBSvc.myDocumentTypesListAsync();
}
private void ddYear_Loaded(object sender, RoutedEventArgs e)
{
loadYear();
}
private void ddMonth_Loaded(object sender, RoutedEventArgs e)
{
loadMonth();
}
private void ddGridHeight_Loaded(object sender, RoutedEventArgs e)
{
loadGridHeight();
}
private void ddPageSize_Loaded(object sender, RoutedEventArgs e)
{
loadPageSize();
}
void showError(string localError)
{
}
}
}
17. Then compile and run the project
18. Be sure to add .xap and .xaml MIME types after the deployment into IIR
19. Now, if we run ImageViewerTestPage.aspx from the browser, we will see the Silverlight Application Interface that we created
Comments
Post a Comment